Below mentioned are some of the core upgrades done as a part of Java 8 release. Just go through them quickly, we will explore them in details later.
- Enhanced Productivity by providing Optional Classes feature, Lamda Expressions, Streams etc.
- Ease of Use
- Improved Polyglot programming. A Polyglot is a program or script, written in a form which is valid in multiple programming languages and it performs the same operations in multiple programming languages. So Java now supports such type of programming technique.
- Improved Security and performance.
What is JVM?
Java virtual Machine(JVM) is a virtual Machine that provides runtime environment to execute java byte code. The JVM doesn't understand Java typo, that's why you compile your
*.java
files to obtain *.class
files that contain the bytecodes understandable by the JVM.
JVM control execution of every Java program. It enables features such as automated exception handling, Garbage-collected heap.
JVM Architecture
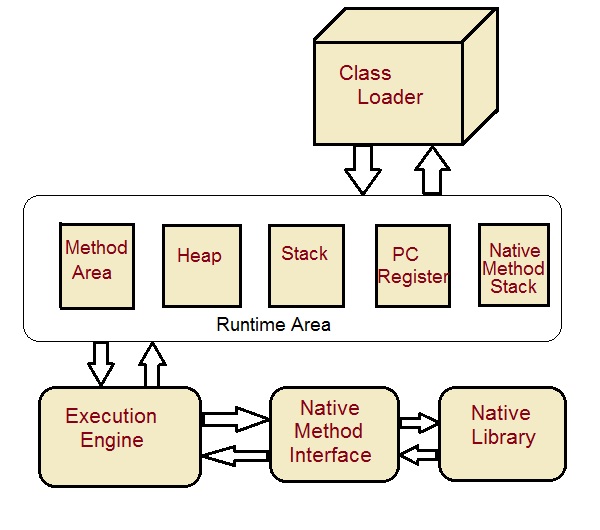
Class Loader : Class loader loads the Class for execution.
Method area : Stores pre-class structure as constant pool.
Heap : Heap is in which objects are allocated.
Stack : Local variables and partial results are store here. Each thread has a private JVM stack created when the thread is created.
Program register : Program register holds the address of JVM instruction currently being executed.
Native method stack : It contains all native used in application.
Executive Engine : Execution engine controls the execute of instructions contained in the methods of the classes.
Native Method Interface : Native method interface gives an interface between java code and native code during execution.
Native Method Libraries : Native Libraries consist of files required for the execution of native code.
Difference between JDK and JRE
JRE : The Java Runtime Environment (JRE) provides the libraries, the Java Virtual Machine, and other components to run applets and applications written in the Java programming language. JRE does not contain tools and utilities such as compilers or debuggers for developing applets and applications.
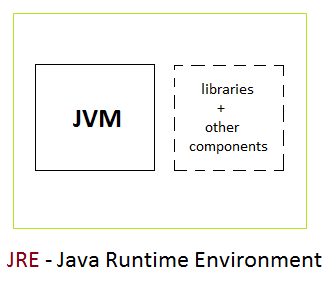
JDK : The JDK also called Java Development Kit is a superset of the JRE, and contains everything that is in the JRE, plus tools such as the compilers and debuggers necessary for developing applets and applications.
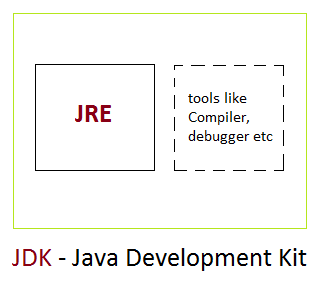
Now we can compile small Hello.java how it will works we can see in below at Run time.
class Hello
{
public static void main(String[] args)
{
System.out.println ("Hello World program");
}
}
class : class keyword is used to declare classes in Java
public : It is an access specifier. Public means this function is visible to all.
static : static is again a keyword used to make a function static. To execute a static function you do not have to create an Object of the class. The main() method here is called by JVM, without creating any object for class.
void : It is the return type, meaning this function will not return anything.
main : main() method is the most important method in a Java program. This is the method which is executed, hence all the logic must be inside the main() method. If a java class is not having a main() method, it causes compilation error.
String[] args : This represents an array whose type is String and name is args. We will discuss more about array in Java Array section.
System.out.println : This is used to print anything on the console like printf in C language.
Now let us see What happens at Runtime
After writing your Java program, when you will try to compile it. Compiler will perform some compilation operation on your program.
Once it is compiled successfully byte code(.class file) is generated by the compiler.
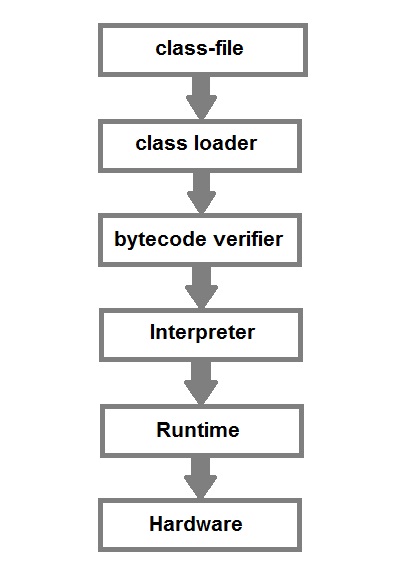
After compiling when you will try to run the byte code(.class file), the following steps are performed at runtime:-
- Class loader loads the java class. It is subsystem of JVM Java Virtual machine.
- Byte Code verifier checks the code fragments for illegal codes that can violate access right to the object.
- Interpreter reads the byte code stream and then executes the instructions, step by step.
When to use Inheritance and Aggregation?
When you need to use property and behavior of a class without modifying it inside your class. In such case Aggregation is a better option. Whereas when you need to use and modify property and behavior of a class inside your class, its best to use Inheritance.
Q. What is Composition in java?
Composition is restricted form of Aggregation. Composition can be described as when one class which owns the other class, is destroyed then the other class cannot exist meaningfully without it. For example a class Car cannot exist without Engine.
Aggregation (HAS-A)
HAS-A relationship is based on usage, rather than inheritance. In other words, class A has-a relationship with class B, if code in class A has a reference to an instance of class B.
Example
class Student
{
String name;
Address ad;
}
Here you can say that Student has-a Address.
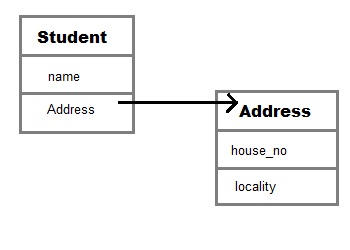
Student class has an instance variable of type Address. Student code can use Address reference to invoke methods on the Address, and get Address behavior.
Aggregation allow you to design classes that follow good Object Oriented practices. It also provide code re usability.
Example of Aggregation
class Author
{
String authorName;
int age;
String place;
Author(String name,int age,String place)
{
this.authorName=name;
this.age=age;
this.place=place;
}
public String getAuthorName()
{
return authorName;
}
public int getAge()
{
return age;
}
public String getPlace()
{
return place;
}
}
class Book
{
String name;
int price;
Author auth;
Book(String n,int p,Author at)
{
this.name=n;
this.price=p;
this.auth=at;
}
public void showDetail()
{
System.out.println("Book is"+name);
System.out.println("price "+price);
System.out.println("Author is "+auth.getAuthorName());
}
}
class Test
{
public static void main(String args[])
{
Author ath=new Author("Me",22,"India");
Book b=new Book("Java",550,ath);
b.showDetail();
}
}
OUTPUT:
Book is Java.
price is 550.
Author is me.
When to use Abstract Methods & Abstract Class?
Abstract methods are usually declared where two or more subclasses are expected to do a similar thing in different ways through different implementations. These subclasses extend the same Abstract class and provide different implementations for the abstract methods.
Abstract classes are used to define generic types of behaviors at the top of an object-oriented programming class hierarchy, and use its subclasses to provide implementation details of the abstract class.
Points to Remember
- Abstract classes are not Interfaces. They are different, we will study this when we will study Interfaces.
- An abstract class may or may not have an abstract method. But if any class has even a single abstract method, then it must be declared abstract.
- Abstract classes can have Constructors, Member variables and Normal methods.
- Abstract classes are never instantiated.
- When you extend Abstract class with abstract method, you must define the abstract method in the child class, or make the child class abstract.
Abstract class
If a class contain any abstract method then the class is declared as abstract class. An abstract class is never instantiated. It is used to provide abstraction. Although it does not provide 100% abstraction because it can also have concrete method.
Abstract method
Method that are declared without any body within an abstract class are called abstract method. The method body will be defined by its subclass. Abstract method can never be final and static. Any class that extends an abstract class must implement all the abstract methods declared by the super class.
Can Abstract class have Constructor ?
Yes, an abstract class can have a constructor. Consider this:
abstract class Product {
int multiplyBy;
public Product( int multiplyBy ) {
this.multiplyBy = multiplyBy;
}
public int mutiply(int val) {
return multiplyBy * val;
}
}
class TimesTwo extends Product {
public TimesTwo() {
super(2);
}
}
class TimesWhat extends Product {
public TimesWhat(int what) {
super(what);
}
}
The superclass
Product
is abstract and has a constructor. The concrete class TimesTwo
has a constructor that just hardcodes the value 2. The concrete class TimesWhat
has a constructor that allows the caller to specify the value.
Abstract constructors will frequently be used to enforce class constraints or invariants such as the minimum fields required to setup the class.
Example 2 :
Conclusion:
Example 2 :
abstract class Car {
// Determine how many years a car will last based on other components
int lifeTimeInYears;
float price;
public Car(float price) {
// Assuming you could calculate the longevity based on price;
if (price > 50000) {
lifeTimeInYears = 15;
}
else {
lifeTimeInYears = 10;
}
}
public int getLifeTimeInYears() {
return lifeTimeInYears;
}
}
class SportsCar extends Car {
public SportsCar(float price) {
super(price);
}
}
class CommuterCar extends Car {
public CommuterCar(float price) {
super(price);
}
}
public class Test {
public static void main(String[] args) {
SportsCar sportsCar = new SportsCar(150000);
sportsCar.getLifeTimeInYears(); // Value is 15
CommuterCar commuterCar = new CommuterCar(15000);
commuterCar.getLifeTimeInYears(); // Value is 10
}
}
Conclusion:
A constructor in Java doesn't actually "build" the object, it is used to initialize fields.
Imagine that your abstract class has fields x and y, and that you always want them to be initialized in a certain way, no matter what actual concrete subclass is eventually created. So you create a constructor and initialize these fields.
Now, if you have two different subclasses of your abstract class, when you instantiate them their constructors will be called, and then the parent constructor will be called and the fields will be initialized.
If you don't do anything, the default constructor of the parent will be called. However, you can use the super keyword to invoke specific constructor on the parent class.
No comments:
Post a Comment